Classes | |
class | ev3_control::Ev3ColorController |
class | ev3_control::Ev3GyroController |
class | ev3_control::Ev3InfraredController |
class | ev3_control::Ev3TouchController |
class | ev3_control::Ev3UltrasonicController |
Detailed Description
Ev3ColorController
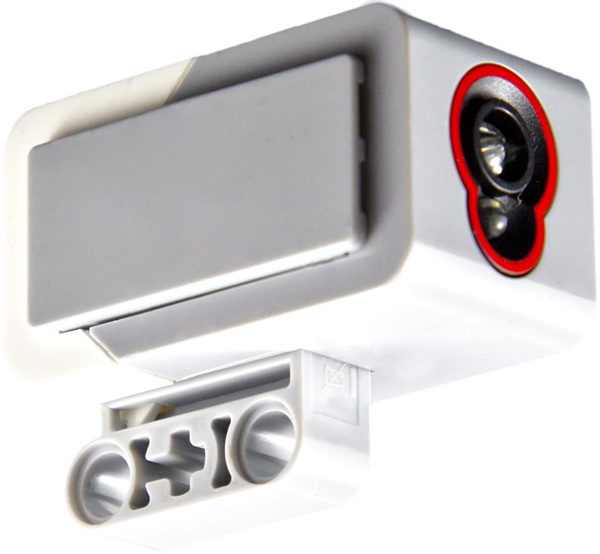
Function
This controller is used to read the standard EV3 Color Sensor
Parameters
publish_rate
The rate which the controller should use to publish its messages.
port
Specifies the EV3 port name.
mode
The mode parameter sets the mode for the color sensor. If not set, it will use rgb_raw.
The following modes are possible for the sensor:
- rgb_raw
'rgb_raw' mode uses the RGB_RAW mode of this sensor. It will output the color information in RGB in a std_msgs::ColorRGBA.msg topic. - color
'color' mode publishes the a number for the following recognized colors into a std_msgs::UInt8 topic
Value Color 0 none 1 black 2 blue 3 green 4 yellow 5 red 6 white 7 brown - ambient
'ambient' mode measures the ambient light and publishes into a sensor_msgs::Illuminance topic reflect
'reflect' mode measures the reflected light from an obstacle and publishes into a sensor_msgs::Illuminance topic- Warning
- Currently ambient and reflect publish the direct sensor value (percent) in percent - this will change so that it fits the output value in the message or the message type will be changed when it is found to be not right.
- Todo:
- check how to calculate LUX value for message or change message type
frame_id
The frame_id used in the message
topic_name
The topic name used for the output topic.
Ev3GyroController
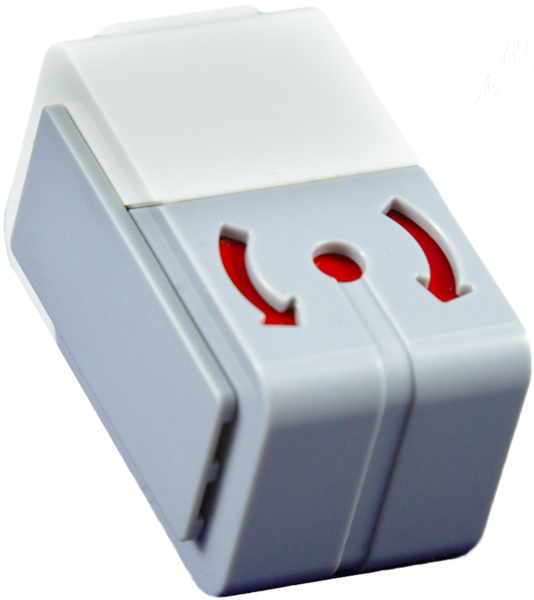
Function
This controller is used to read the standard EV3 Gyro Sensor
Parameters
publish_rate
The rate the controller should use to publish its messages.
port
Specifies the EV3 port name.
mode
The mode parameter sets the mode for the gyro sensor. If not set, it will use angle.
- rate publishes the turn rate into a sensor_msgs::Imu topic (z-axis).
- angle publishes the turn rate into a sensor_msgs::Imu topic (z-axis).
- rate&angle publishes both turn and angle into a sensor_msgs::Imu topic (z-axis).
- Warning
- rate&angle has, according to the EV3Dev manual, an instability. If the angle reaches 32767 or -32768 it will be stuck there. This is a sensor issue. Using this mode is not recommended.
frame_id
The frame_id used in the message
topic_name
The topic name used for the topic.
Ev3InfraredController
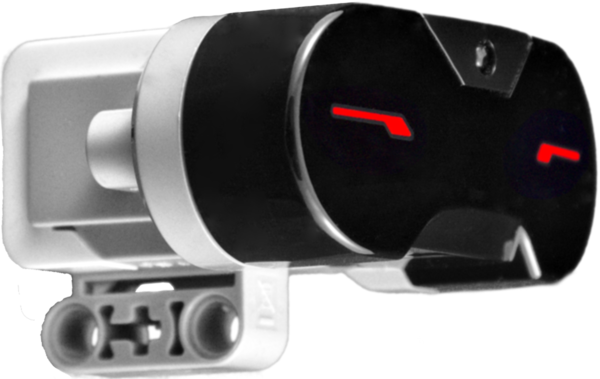
Function
This controller is used to read the standard EV3 Infrared Sensor
Parameters
publish_rate
The rate which the controller should use to publish its messages.
port
Specifies the EV3 port name.
mode
- proximity publishes the distance of the sensor to a reflective surface.
- seek publishes four topics containing the heading and the distance value to ir-remotes in beacon mode for each remote channel
- remote publishes the four remote channels of the ir-sensor to four different topics from type sensor_msgs::Joy
The buttons in the joy topic correlate to the following array element:Element Button 0 red up 1 red down 2 blue up 3 blue down 4 beacon
topic_name
is the topic name used for the output topic or if there are multiple existing topics for the mode like for seek and remote. The topic_name for each topic gets an appending number starting from 0 (e.g. topic_name0).
frame_id
is used in modes publishing a message with an header to set the frame_id for the sensor.
Ev3TouchController
Ev3UltrasonicController
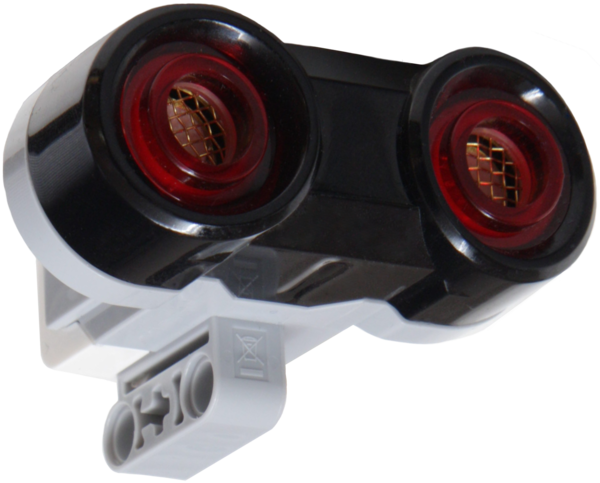
Function
This controller is used to read the standard EV3 Ultrasonic Sensor
Parameters
publish_rate
The rate the controller should use to publish its messages.
port
Specifies the EV3 port name.
mode
- distance publishes the measured distance into a sensor_msgs::Range topic
- listen publishes into a std_msgs::Boolean topic, when it detects another Ultrasonic Sensor or a loud noise (like a clap) the value is true otherwise false.
- max_range sets the maximum range of values taken into account if a value is bigger it will be replaced by infinity.
The maximum value for this parameter is 2.5 because the value 2.550 is the sensors error condition. - min_range sets the minimum range of values taken into account if a value is smaller it will be replaced by negative infinity.
- max_range sets the maximum range of values taken into account if a value is bigger it will be replaced by infinity.